Domain-Driven Design (DDD) Demystified
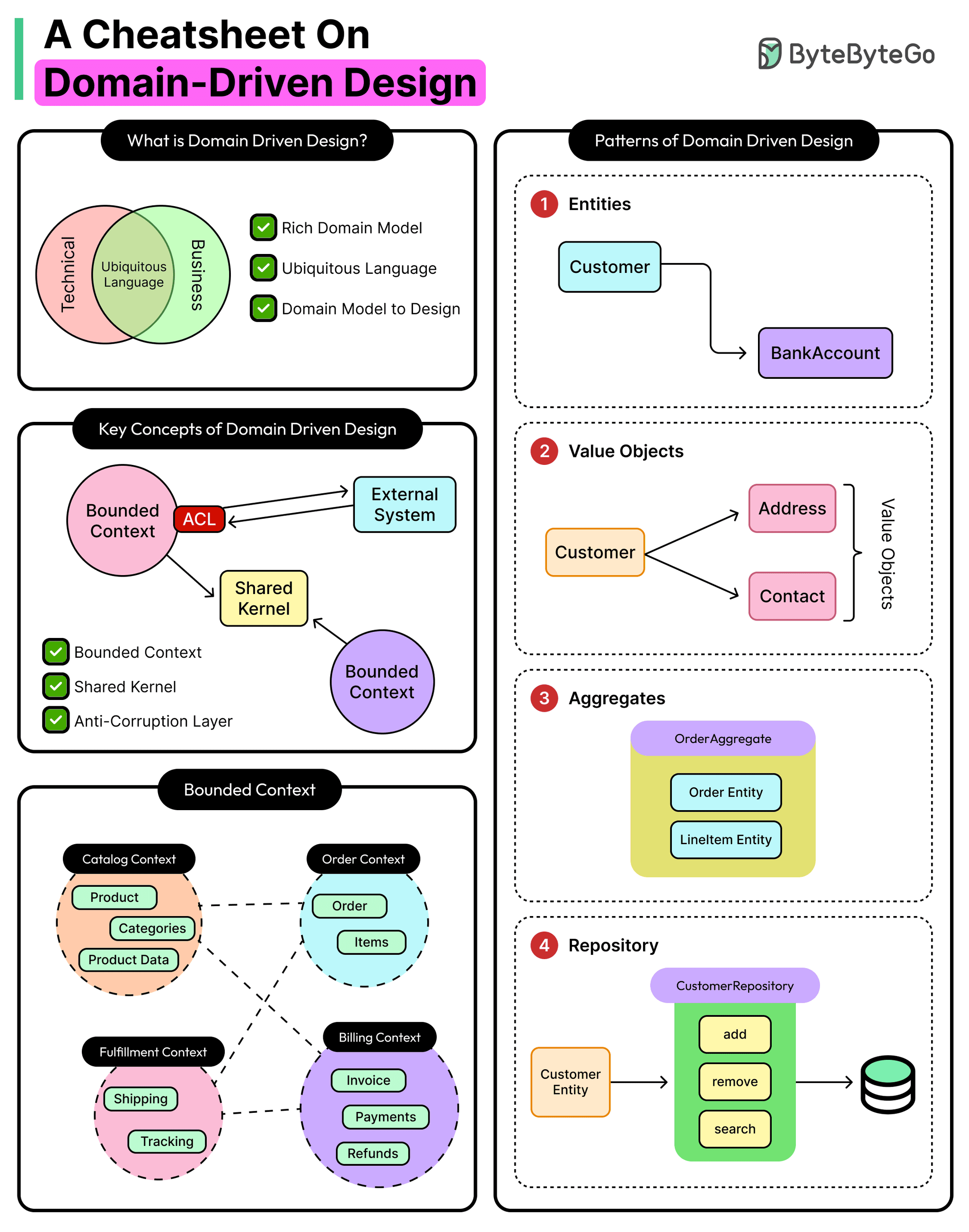
Domain-Driven Design (DDD) is a software development approach that aligns complex systems with business domains by emphasizing clear communication, modular design, and strategic patterns. It focuses on domain modeling, bounded contexts, and ubiquitous language to bridge the gap between technical and business stakeholders. The article explains core DDD concepts, tactical patterns, and practical implementation strategies.
Core Technical Concepts/Technologies
- Domain-Driven Design (DDD)
- Bounded Contexts
- Ubiquitous Language
- Entities & Value Objects
- Aggregates & Repositories
- Domain Events
- Hexagonal (Ports & Adapters) Architecture
Main Points
-
Strategic DDD: Focuses on high-level domain modeling and organizational alignment.
- Bounded Contexts: Explicitly define logical boundaries for domain models to avoid ambiguity.
- Ubiquitous Language: Shared terminology between developers and domain experts to reduce miscommunication.
-
Tactical DDD: Implements domain models with technical building blocks.
- Entities: Objects with unique identities (e.g.,
User
with an ID). - Value Objects: Immutable objects defined by attributes (e.g.,
Address
). - Aggregates: Clusters of related objects treated as a single unit (e.g.,
Order
and itsOrderItems
). - Repositories: Persistence mechanisms for aggregates (e.g.,
OrderRepository
). - Domain Events: Capture state changes (e.g.,
OrderPlacedEvent
).
- Entities: Objects with unique identities (e.g.,
-
Architectural Patterns:
- Hexagonal Architecture isolates domain logic from infrastructure (e.g., databases, UIs).
- CQRS (Command Query Responsibility Segregation) separates read/write operations for scalability.
Technical Specifications & Code Examples
- Aggregate Root Example:
public class Order { // Aggregate Root private String orderId; private List<OrderItem> items; // Enforce invariants (e.g., no empty orders) }
- Domain Event Example:
public class OrderPlacedEvent { private String orderId; private LocalDateTime timestamp; }
Key Takeaways
- Align with Business Goals: DDD prioritizes domain logic over technical implementation.
- Modularize with Bounded Contexts: Decouple subsystems to manage complexity.
- Leverage Tactical Patterns: Use entities, aggregates, and events to model behavior accurately.
- Adopt Hexagonal Architecture: Keep domain logic independent of external systems.
- Iterate on Ubiquitous Language: Continuously refine terminology with domain experts.
Limitations & Caveats
- Overhead: DDD adds complexity for simple CRUD applications.
- Expertise Dependency: Requires collaboration with domain experts.
- Learning Curve: Tactical patterns demand deep understanding of object modeling.
- Scalability: CQRS introduces eventual consistency challenges.
Further Exploration
- Event Sourcing for auditability.
- Microservices alignment with bounded contexts.
- DDD in legacy system modernization.
Most software doesn’t break because of syntax errors or flawed if-else logic.
This article was originally published on ByteByteGo
Visit Original Source